XSS (Cross-Site Scripting)
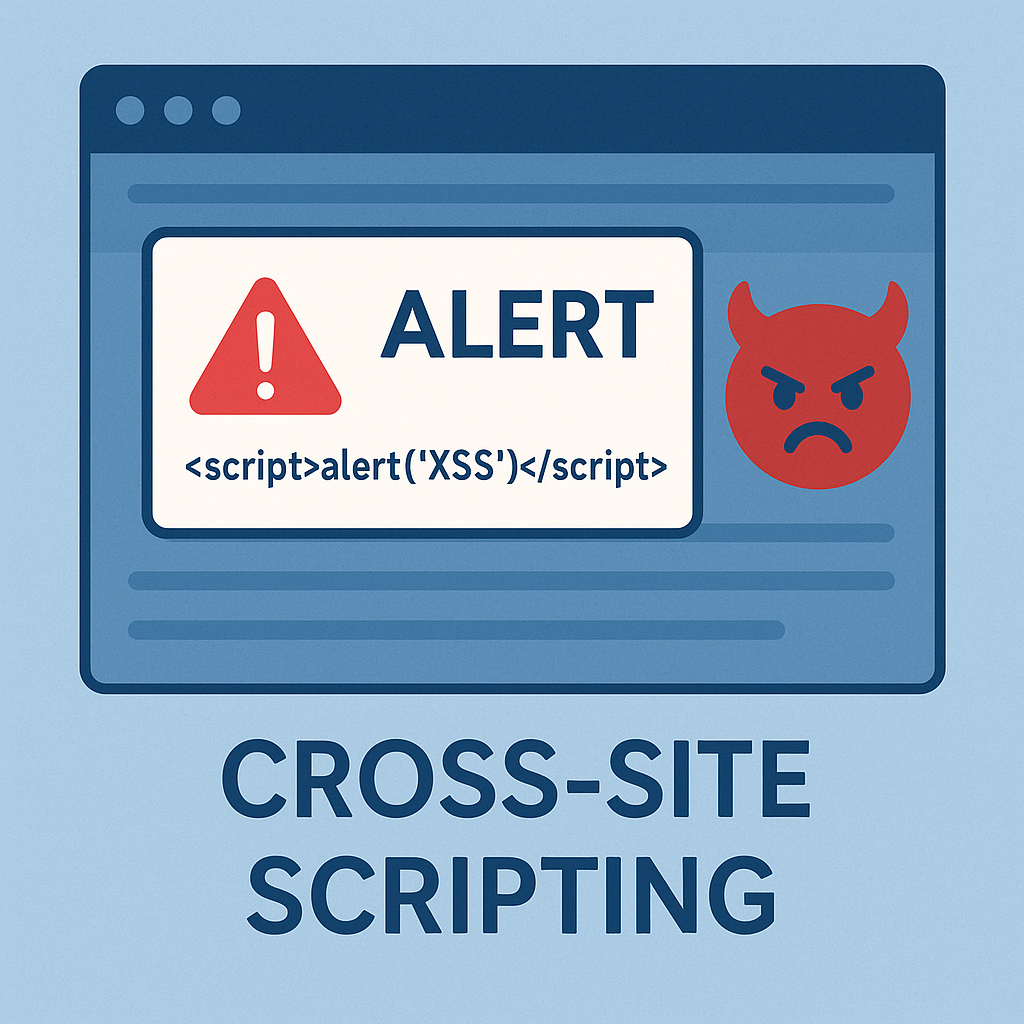
Imagine you visit your favorite website, and suddenly a weird pop-up shows up, or worse—your account is used without your knowledge. You didn't click anything shady. What happened?
👉 That could be Cross-Site Scripting (XSS) — a sneaky trick hackers use to run malicious code on web pages.
👀 XSS can:
-
Steal your login info
-
Hijack your browser session
-
Spread malware
XSS (Cross-Site Scripting) is a security vulnerability that allows attackers to inject malicious scripts into web pages viewed by others. These scripts usually run in the victim's browser and can steal data, hijack sessions, redirect users, or deface websites. XSS happens when a website includes untrusted data in its web pages without proper validation or escaping.
Types of XSS (with Examples)
1. Stored XSS (Persistent XSS):
This happens when the malicious script is saved on the web server, such as in a database, and shown to users later.
Example:
A user posts a comment like:
<script>alert('This site is vulnerable to Stored XSS');</script>
If the website stores and displays this comment without sanitizing it, everyone who visits the page will see the alert popup.
2. Reflected XSS:
This occurs when the malicious script is part of a URL or form input, and the server immediately reflects it in the response.
Example:
A link like:
https://example.com/search?q=<script>alert('Reflected XSS')</script>
If the server prints the value of q directly into the HTML without encoding, the script will run in the victim's browser when they click the link.
3. DOM-Based XSS:
This type of XSS happens entirely in the browser, through insecure client-side JavaScript that reads from the URL or user input and writes to the page without sanitizing it.
Example:
A page has a script like:
document.getElementById("output").innerHTML = location.hash;
If the user opens a URL like:
https://example.com/#<script>alert('DOM XSS')</script>,
the script will execute in their browser.
Real-World Case Studies
1. MySpace Worm by Samy (2005) — Stored XSS
Samy Kamkar created a worm using stored XSS on MySpace. He added a script to his profile that would copy itself to anyone who visited it. Once someone viewed his profile, their profile was infected too, and they automatically added Samy as a friend. It spread to over a million users in under 24 hours.
2. British Airways Credit Card Theft (2018) — DOM-Based XSS
Hackers used a technique similar to DOM-based XSS to inject JavaScript into British Airways' payment page. The script captured user inputs such as credit card details and sent them to the attackers. Around 380,000 card transactions were compromised. The attackers used a method similar to DOM-based injection, manipulating JavaScript on the client side.
3. eBay Listings (Multiple Incidents) — Stored XSS
Attackers inserted malicious JavaScript into product descriptions on eBay. The scripts would run when a buyer viewed the item page, stealing session cookies or redirecting users to fake login pages. Since eBay stored and displayed the descriptions, this was a stored XSS attack.
4. PayPal Redirect (2006) — Reflected XSS
PayPal had a reflected XSS vulnerability where attackers could trick users into clicking a PayPal link with embedded JavaScript. The script ran immediately and redirected users to a phishing site, even though the domain looked trustworthy. This is a classic case of reflected XSS.
5. Google Search (Old Example) — Reflected XSS
In early versions, Google had a search page that didn't properly filter special characters. Attackers could craft URLs that caused JavaScript to execute in users' browsers when they clicked on search result links. This was a reflected XSS issue.
Summary
XSS is a serious threat in web security. The three main types are:
-
Stored XSS: Script is saved on the server and affects every user who loads the page.
-
Reflected XSS: Script comes from the user input (like a URL) and is reflected back immediately.
-
DOM-Based XSS: Script runs entirely in the browser due to insecure client-side JavaScript.
To protect against XSS, developers should always sanitize inputs, encode outputs, and use features like Content Security Policy (CSP).
🔐 Cross-Site Scripting (XSS) Protection
1. Know Your Enemy: Types of XSS
Before defending against XSS, understand the three major types:
-
Stored XSS: The malicious script is permanently stored on the server (e.g., in a database or forum post) and served to victims.
-
Reflected XSS: The script is reflected off the server via a request (e.g., URL parameters) and executed immediately.
-
DOM-based XSS: Happens entirely on the client side, where JavaScript modifies the DOM using untrusted user input.
2. Validate Input and Encode Output
Input Validation
Always validate user input on both the client and server sides:
Only allow expected input (e.g., numbers for age, alphabets for names).
Use whitelisting techniques rather than trying to blacklist bad inputs.
Don't rely solely on JavaScript validation—server-side checks are crucial.
3, Output Encoding (Context-Aware Escaping)
- Encode untrusted data before inserting it into HTML, JavaScript, CSS, or URLs:
- Escape HTML-specific characters like <, >, ", and ' to prevent script injection.
- In JavaScript, use safe serialization methods like JSON.stringify().
- For attributes or CSS contexts, use the right encoding method based on where the data will be placed.
- Never insert untrusted data into innerHTML. Use safer DOM APIs like textContent.
3. Use Security Headers
Content Security Policy (CSP)
CSP is one of the strongest defences against XSS:
It restricts the sources from which scripts can be executed.A well-configured CSP blocks inline scripts and unauthorized domains.
Example: Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted.cdn.com
X-XSS-Protection
This is a legacy browser feature that prevents some reflected XSS attacks:
Example: X-XSS-Protection: 1; mode=blockHowever, modern browsers have deprecated this; CSP is preferred.
4. Avoid Unsafe JavaScript Patterns
Certain functions are dangerous if misused with untrusted input:
-
Avoid using innerHTML, document.write, eval, setTimeout, or setInterval with strings that include user data.
-
Use textContent, setAttribute, and createElement() to safely build DOM content.
5. Perform Security Testing Regularly
Automated Testing
Use tools like:
OWASP ZAP– Free and open-source web scanner.
Burp Suite– Professional suite for advanced XSS testing.
Static code analyzerslike ESLint (with security plugins) or SonarQube.
Manual Testing
Manually test forms, URLs, headers, and APIs:
Use test payloads like <script>alert('XSS')</script> or <img src=x onerror=alert('XSS')>Check if responses reflect unescaped input.
6. Leverage Secure Frameworks
Modern frameworks offer built-in protections:
-
React escapes data by default in JSX.
-
Angular automatically sanitizes HTML, CSS, and URL bindings.
-
Vue escapes output unless v-html is used (which should be avoided or carefully handled).
Still, never bypass these protections unless absolutely necessary—and always sanitize manually if you do.
7. Keep Everything Updated
-
Regularly patch and update your frameworks, libraries, and dependencies.
-
Use tools like npm audit, yarn audit, Snyk, or Dependabot to stay informed about vulnerabilities.
Final Advice
Preventing XSS is not about a single control—it's about layered defense. Combine:
-
Proper input validation,
-
Output encoding,
-
Secure coding practices,
-
HTTP security headers,
-
Testing and monitoring,
-
And keeping all components up to date.
A single overlooked innerHTML or a missing CSP rule can open the door to serious security risks.
Multiple choice questions (MCQs) on Cross-Site Scripting (XSS)
1. What is the main goal of a Cross-Site Scripting (XSS) attack?
A. To crash the server
B. To inject malicious scripts into web pages
C. To overload the database
D. To send spam emails
Answer: B
Explanation: XSS allows attackers to inject and execute malicious scripts in the context of users' browsers.
2. Which language is most commonly used in XSS attacks?
A. Python
B. PHP
C. JavaScript
D. SQL
Answer: C
Explanation: JavaScript is the primary language used in XSS attacks because it runs in the browser.
3. In which type of XSS is the payload permanently stored on the server?
A. Reflected XSS
B. DOM-Based XSS
C. Stored XSS
D. SQL Injection
Answer: C
Explanation: Stored XSS (Persistent XSS) saves the script in the server (e.g., in a database) and delivers it to users.
4. Which XSS type involves manipulating the web page entirely on the client-side using JavaScript?
A. Reflected XSS
B. Stored XSS
C. DOM-Based XSS
D. CSRF
Answer: C
Explanation: DOM-Based XSS occurs when the page's JavaScript reads and uses user-controlled data insecurely.
5. What is a common result of a successful XSS attack?
A. The server crashes
B. The database becomes read-only
C. User session tokens are stolen
D. User passwords are reset
Answer: C
Explanation: XSS is commonly used to steal cookies and session tokens to impersonate users.
6. Which of the following is a valid prevention technique against XSS?
A. Running antivirus on the server
B. Using stronger passwords
C. Escaping user input before displaying it
D. Using SQL queries with parameters
Answer: C
Explanation: Escaping or sanitizing user input before rendering it in HTML prevents XSS.
7. What does CSP stand for in the context of XSS protection?
A. Code Security Protocol
B. Content Security Policy
C. Client Script Protection
D. Cookie Security Plugin
Answer: B
Explanation: CSP (Content Security Policy) restricts the sources from which scripts can be loaded and executed.
8. What is typically the entry point for an XSS attack?
A. Admin panel
B. Login form
C. User input fields (e.g., forms, URLs)
D. FTP access
Answer: C
Explanation: XSS often starts with untrusted input fields like search boxes, comments, or query strings.
9. A script is injected into a URL and immediately reflected in the server response. What type of XSS is this?
A. Stored XSS
B. Reflected XSS
C. DOM-Based XSS
D. Remote File Inclusion
Answer: B
Explanation: Reflected XSS happens when the script is in the URL or request and reflected right back in the response.
10. Which of these tools is commonly used to detect XSS vulnerabilities?
A. Nmap
B. Wireshark
C. Burp Suite
D. Netcat
Answer: C
Explanation: Burp Suite is a popular tool for testing web application security, including XSS vulnerabilities.
11. Which HTTP header can help reduce XSS attacks by controlling script sources?
A. X-Content-Type-Options
B. Content-Security-Policy
C. X-Frame-Options
D. Access-Control-Allow-Origin
Answer: B
Explanation: Content-Security-Policy defines which sources are trusted for executing scripts, reducing XSS risk.
12. What is a common effect of failing to encode output properly in web apps?
A. CSRF attacks
B. SQL Injection
C. XSS attacks
D. DNS poisoning
Answer: C
Explanation: Improper output encoding can allow scripts to be injected into the rendered web page (XSS).
13. Which function should you avoid using due to XSS risks?
A. console.log()
B. alert()
C. document.write()
D. parseInt()
Answer: C
Explanation: document.write() can introduce DOM-based XSS when used with untrusted input.
14. Which of these is an XSS payload?
A. 1=1--
B. <script>alert('XSS')</script>
C. DROP TABLE users;
D. ../etc/passwd
Answer: B
Explanation: This is a classic script injection payload used to test for XSS.
15. In which part of a web page is XSS most dangerous?
A. Inside comments
B. Inside HTML tags
C. Inside <script> or <img onerror>
D. Inside CSS files
Answer: C
Explanation: Scripts injected directly into tags or script blocks are most dangerous.
16. What does the "onerror" attribute commonly enable in XSS payloads?
A. Styling pages
B. Redirecting domains
C. Executing scripts on broken images
D. Disabling cookies
Answer: C
Explanation: Attackers often use onerror in image tags to execute JavaScript.
17. Which of the following best defines DOM-based XSS?
A. Injection stored on server
B. Immediate reflection by server
C. Code executed via client-side scripts
D. Code executed on the DNS layer
Answer: C
Explanation: DOM-based XSS relies on insecure JavaScript handling input on the client side.
18. A website uses innerHTML to show user input. What should you use instead?
A. outerHTML
B. document.write()
C. textContent
D. value
Answer: C
Explanation: textContent safely adds text to the page without executing scripts.
19. What does OWASP stand for?
A. Online Web App Sec Policy
B. Open Web Application Security Project
C. Only Web Apps Secure Portal
D. Online Wide Area Sec Program
Answer: B
Explanation: OWASP is a global nonprofit that provides best practices for web app security, including XSS defense.
20. Which of the following is an incorrect way to prevent XSS?
A. Escaping output
B. Validating input
C. Using eval() for output
D. Applying CSP
Answer: C
Explanation: eval() should be avoided as it can execute arbitrary code and lead to XSS.
21. What is the most effective place to filter input to prevent XSS?
A. At the database level
B. Before it reaches the user's browser
C. After it is stored
D. On the CDN layer
Answer: B
Explanation: Filtering input or escaping output before it reaches the user is critical to stopping XSS.
22. Which user action could trigger a stored XSS attack?
A. Searching on a site
B. Submitting a contact form
C. Posting a comment with a script
D. Clicking a broken link
Answer: C
Explanation: Stored XSS often uses comment fields or user-generated content to persist malicious code.
23. Which of the following HTML tags is often misused in XSS payloads?
A. <div>
B. <b>
C. <script>
D. <p>
Answer: C
Explanation: <script> tags directly execute JavaScript, making them prime targets for XSS.
24. What is the main reason XSS is dangerous in session management?
A. It resets user passwords
B. It bypasses firewalls
C. It steals session cookies
D. It encrypts session tokens
Answer: C
Explanation: XSS can be used to steal session cookies and hijack authenticated sessions.
25. What role does the Same-Origin Policy play in XSS?
A. It blocks script execution
B. It allows all scripts to run
C. It isolates data between domains
D. It prevents CSS rendering
Answer: C
Explanation: The Same-Origin Policy limits access between pages from different domains. XSS can bypass this if a script runs in a trusted context.
26. A user opens an email link that redirects to a URL with script in the query string. This is an example of:
A. SQL Injection
B. Stored XSS
C. Reflected XSS
D. Buffer Overflow
Answer: C
Explanation: Reflected XSS often uses malicious URLs to deliver the attack.
27. Which type of XSS is hardest to detect with traditional scanners?
A. Stored
B. Reflected
C. DOM-Based
D. Server-Side
Answer: C
Explanation: DOM-based XSS happens entirely in the browser and often escapes server-side scanners.
28. How can cookies be protected from being stolen via XSS?
A. Enable HttpOnly flag
B. Use larger cookies
C. Store cookies in localStorage
D. Block JavaScript
Answer: A
Explanation: Cookies marked as HttpOnly cannot be accessed by JavaScript, making them safe from XSS theft.
29. What is the purpose of input validation in XSS prevention?
A. To improve speed
B. To filter data before saving
C. To check file types
D. To limit bandwidth
Answer: B
Explanation: Input validation prevents malicious code from being saved or reflected.
30. Which attack could chain with XSS to escalate privileges?
A. CSRF
B. DDoS
C. Port scanning
D. Brute force
Answer: A
Explanation: XSS combined with CSRF (Cross-Site Request Forgery) can perform actions on behalf of authenticated users.
31. What does innerHTML = userInput risk if userInput is not sanitized?
A. Slower load times
B. XSS execution
C. Form misalignment
D. Image rendering issues
Answer: B
Explanation: Using innerHTML with unsanitized input can allow XSS scripts to execute.
32. A secure input sanitization function should:
A. Allow all HTML tags
B. Remove script content
C. Accept JavaScript input
D. Encode SQL syntax
Answer: B
Explanation: The function should strip or neutralize script tags and dangerous content.
33. Which of these is not a client-side XSS example?
A. Reflected XSS
B. Stored XSS
C. DOM-Based XSS
D. SQL Injection
Answer: D
Explanation: SQL injection affects the database; it is not an XSS attack.
34. What is the role of sanitize-html in Node.js?
A. Encrypt passwords
B. Remove XSS content from HTML
C. Generate scripts
D. Minify code
Answer: B
Explanation: sanitize-html is a library that helps clean user input by removing XSS-prone content.
35. What is a real-world danger of an XSS worm?
A. It infects a user's hard drive
B. It spreads XSS automatically across users
C. It deletes web servers
D. It sends spam emails
Answer: B
Explanation: An XSS worm like the MySpace Samy worm spreads malicious scripts automatically between users.